ラズパイデスクトップでPyQt5入門(21)Painting in PyQt5(3)
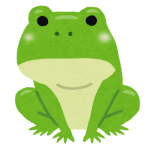
こんばんは。湿度が高いとテンション下がる系の國松です。
参考サイト
ZetCode PyQt5 tutorial
【PythonでGUI】PyQt5 -始めの一歩-
5.QBrush(塗りつぶし)
QBrushは長方形、楕円、多角形などのグラフィック形状の背景をペイントするために使用します。
ブラシには定義済みのブラシ、グラデーション、テクスチャパターンの3種類があります。
今回は背景が異なる9個の長方形を描画していきたいと思います。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 | #!/usr/bin/python3 #-*-coding:utf-8-*- """ This example draws nine rectangles in different brush styles """ from PyQt5.QtWidgets import QWidget,QApplication from PyQt5.QtGui import QPainter,QBrush from PyQt5.QtCore import Qt import sys class Example(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): self.setGeometry(300,300,355,280) self.setWindowTitle('Brushes') self.show() def paintEvent(self,e): qp = QPainter() qp.begin(self) self.drawBrushes(qp) qp.end() def drawBrushes(self,qp): brush = QBrush(Qt.SolidPattern) qp.setBrush(brush) qp.drawRect(10,15,90,60) brush = QBrush(Qt.Dense1Pattern) qp.setBrush(brush) qp.drawRect(130,15,90,60) brush = QBrush(Qt.Dense2Pattern) qp.setBrush(brush) qp.drawRect(250,15,90,60) brush.setStyle(Qt.DiagCrossPattern) qp.setBrush(brush) qp.drawRect(10,105,90,60) brush.setStyle(Qt.Dense5Pattern) qp.setBrush(brush) qp.drawRect(130,105,90,60) brush.setStyle(Qt.Dense6Pattern) qp.setBrush(brush) qp.drawRect(250,105,90,60) brush.setStyle(Qt.HorPattern) qp.setBrush(brush) qp.drawRect(10,195,90,60) brush.setStyle(Qt.VerPattern) qp.setBrush(brush) qp.drawRect(130,195,90,60) brush.setStyle(Qt.BDiagPattern) qp.setBrush(brush) qp.drawRect(250,195,90,60) if __name__ == '__main__': app = QApplication(sys.argv) ex = Example() sys.exit(app.exec_()) |
brush.QVrush(Qt.SolidPattern) ブラシオブジェクトを定義
qp.setBrush(brush) ペイントオブジェクトにブラシを設定
qp.drawRect(10,15,90,60) 長方形を描画して塗りつぶす
6.Bezier Curve(ベジェ曲線)。
ベジェ曲線とは?
N個の制御点から得られるN-1次曲線の事です。どんな曲線か直ぐにイメージできたあなたはすごい。
ざっくり言えばCG等において滑らかな曲線を描くためによく利用される曲線です。(ざっくりしすぎですみません)
PyQt5のベジエ曲線はQPainterPathで作成できます。QPainterPathは長方形、楕円、線、曲線など多くのグラフィカルなビルディングブロックで構成させるオブジェクトです。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 | #!/usr/bin/python3 #-*-coding:utf-8-*- """ This program draws a bezier curve eith QPainterPath """ from PyQt5.QtWidgets import QWidget, QApplication from PyQt5.QtGui import QPainter,QPainterPath from PyQt5.QtCore import Qt import sys class Example(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): self.setGeometry(300,300,380,250) self.setWindowTitle('Bezier curve') self.show() def paintEvent(self,e): qp = QPainter() qp.begin(self) qp.setRenderHint(QPainter.Antialiasing) self.drawBezierCurve(qp) qp.end() def drawBezierCurve(self,qp): path = QPainterPath() path.moveTo(30,30) path.cubicTo(30,30,200,350,350,30) qp.drawPath(path) if __name__ == '__main__': app = QApplication(sys.argv) ex = Example() sys.exit(app.exec_()) |
Path = QPainterPath() //QPainterPathを使用してベジェ曲線を作成
Path.move(30,30)
Path.cubicto(30,30,200,250,350,30) //30,30が開始点、200,350が制御点、350,30が終了点
draw.path()で描画
今回はここまでになります。それではまた。