ラズパイデスクトップでPyQt5入門(18)Drag and Drop
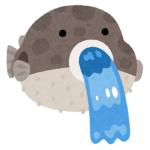
こんばんは。國松です。
今回はPyQt5のドラッグ&ドロップを見て行きたいと思います。
参考サイト
ZetCode PyQt5 tutorial
【PythonでGUI】PyQt5 -始めの一歩-
QDrag
QDragはMIMEベースのドラッグアンドドロップデータ転送のサポートを提供します。
ドラッグアンドドロップ操作のほとんどを処理します。
転送されたデータはQMimeDataオブジェクトに含まれています。
1.Simple drag and drop
QLineEditとQPushButtonを作成します。
QLineEditからプレーンテキストをドラッグしてボタンにドロップするとボタンのラベルが変更されます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 | #!/usr/bin/python3 #-*- coding; utf-8 -*- """ This is a simple drag and drop example """ from PyQt5.QtWidgets import(QPushButton,QWidget,QLineEdit,QApplication) import sys #QPushButtonを継承 class Button(QPushButton): def __init__(self,title,parent): super().__init__(title,parent) #ボタンに対してドロップ操作を可能にする self.setAcceptDrops(True) def dragEnterEvent(self,e): #ドラッグ可能なデータ形式を設定 if e.mimeData().hasFormat('text/plain'): e.accept() else: e.ignore() def dropEvent(self,e): #ドロップしたときにボタンのラベルを変更 self.setText(e.mimeData().text()) class Example(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): edit = QLineEdit('',self) #ドラッグ可能にする edit.setDragEnabled(True) edit.move(30,65) button = Button("Button",self) button.move(190,65) self.setWindowTitle("Simple drag and drop") self.setGeometry(300,300,300,150) if __name__ == '__main__': app = QApplication(sys.argv) ex = Example() ex.show() app.exec_() |
2.Drag and drop a button widget
Buttonウィジェットをドラッグアンドドロップしていきます。
マウスの左ボタンをクリック➡コンソールに「press」と表示
マウスの右ボタンでボタンをドラッグアンドドロップで移動できる。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 | #!/usr/bin/python3 #-*- coding: utf-8 -*- """ In this program,we can press on a button with a left mouse click or drag and drop the button with hthe right mouse click """ from PyQt5.QtWidgets import QPushButton,QWidget,QApplication from PyQt5.QtCore import Qt, QMimeData from PyQt5.QtGui import QDrag import sys class Button(QPushButton): def __init__(self,title,parent): super().__init__(title,parent) def mouseMoveEvent(self,e): #右クリックだけドラッグ&ドロップ可能にする if e.buttons() != Qt.RightButton: return #ドラッグ&ドロップされるデータ形式を代入 mimeData = QMimeData() drag = QDrag(self) drag.setMimeData(mimeData) #ドロップした位置にボタンの左上をセット drag.setHotSpot(e.pos() - self.rect().topLeft()) dropAction = drag.exec_(Qt.MoveAction) def mouPressEvent(self,e): #ボタンが押されたときのボタンの色の変化 super().mousePressEvent(e) #左クリックのときコンソールにpressと表示 if e.button() == Qt.LeftButton: print('press') class Example(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): self.setAcceptDrops(True) self.button = Button('Button',self) self.button.move(100,65) self.setWindowTitle('Click or Move') self.setGeometry(300,300,280,150) def dragEnterEvent(self,e): e.accept() def dropEvent(self,e): #ドラッグ後のマウスの位置にボタンを配置 position = e.pos() self.button.move(position) e.setDropAction(Qt.MoveAction) e.accept() if __name__ == '__main__': app =QApplication(sys.argv) ex = Example() ex.show() app.exec_() |
QPushButtonを継承してButtonクラスを作成
mouseMoveEvent()メソッドとmousePressEvent()メソッドを再実装
if e.buttons() != Qt.RightButton:
return
マウスの右ボタンでのみドラッグアンドドロップを実行できると判断