ラズパイデスクトップでPyQt5入門(16)WidgetsⅡ(1)
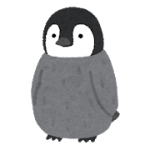
こんにちは。國松です。
今回はWidgetsⅡとなっていますが何のことはありませんPyQtWidgetsの続きです。
QPixmapとQlineEditをみていきたいと思います。
参考サイト
ZetCode PyQt5 tutorial
【PythonでGUI】PyQt5 -始めの一歩-
1.QPixmap
QPixmapは画像の操作に使用されるウィジェットの一つです。
画像を画面に表示するために最適化されています。今回はQPixmapを使用してウィンドウに画像を表示してきたいと思います。表示する画像はpixmap.pyと同じディレクトリに配置してください。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 | #!/usr/bin/python3 #-*-coding:utf-8 """ In this example, we display an image on the window """ from PyQt5.QtWidgets import (QWidget,QHBoxLayout,QLabel,QApplication) from PyQt5.QtGui import QPixmap import sys class Example(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): hbox = QHBoxLayout(self) pixmap = QPixmap("takoyaki.jpg") lbl = QLabel(self) lbl.setPixmap(pixmap) hbox.addWidget(lbl) self.setLayout(hbox) self.move(300,300) self.setWindowTitle('Takoyaki') self.show() if __name__ == '__main__': app = QApplication(sys.argv) ex = Example() sys.exit(app.exec_()) |
pixmap = QPixmap(“redrock.png”)
QPixmapオブジェクト作成します。パラメータにファイル名を指定しています。
lbl = QLabel(self)
lbl.setPixmap(pixmap)
pixmapをQLabelウィジェットに配置します。
2.QlineEdit
QLineEditは1行のプレーンテキストを入力できるウィジェットです。
「元に戻す」「やり直し」「切り取り」「貼り付け」の機能が利用できます。
QLineEditに入力したテキストをラベルに表示していきます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 | #!/usr/bin/python3 #-*-coding:utf-8 -*- """ This example shows text which is entered in a QLineEdit in a QLabel widget """ from PyQt5.QtWidgets import (QWidget,QLabel,QLineEdit,QApplication) import sys class Example(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): #ラベルオブジェクトの作成 self.lbl = QLabel(self) #QlineEditオブジェクトの作成 qle = QLineEdit(self) qle.move(60,100) self.lbl.move(60,40) #QlineEditウィジェットのテキストが変更されたらonChanged()メソッドを呼び出す qle.textChanged[str].connect(self.onChanged) self.setGeometry(300,300,280,170) self.setWindowTitle('QLineEdit') self.show() def onChanged(self,text): #QlineEditに入力したテキストをラベルに設定 self.lbl.setText(text) #ラベルのサイズをテキストの長さに調整 self.lbl.adjustSize() if __name__ == '__main__': app = QApplication(sys.argv) ex = Example() sys.exit(app.exec_()) |