Qtプログラミング – スプレッドシート編集機能 – コピー、削除、切り取り続き
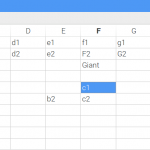
津路です。
今回は、前回実装した機能を利用します。
その前に、Spreadsheetクラスに、コピー、削除関数の宣言を、public slotsとして付け加えます。
1 2 3 4 5 6 7 8 9 10 11 | class Spreadsheet : public QTableWidget { Q_OBJECT public: Spreadsheet(QWidget *parent = 0); public slots: void cut(); void copy(); void del(); void paste(); } |
paste関数の内容です。
大まかには、現在選択中のセル数と、クリップボード上のセル数とを比較して、一致すれば貼り付けます。
前回同様、selectedRangeで選択中のセルを取得します。
各列は、タブ記号で区切られているものとします。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 | void Spreadsheet::paste() { QTableWidgetSelectionRange range = selectedRange(); QString str = QApplication::clipboard()->text(); QStringList rows = str.split('\n'); int numRows = rows.count(); int numColumns = rows.first().count('\t') +1; if(range.rowCount() * range.columnCount() != 1 && (range.rowCount() != numRows || range.columnCount() != numColumns)){ QMessageBox::information(this,tr("SpreadSheet"), tr("The information cannot be pasted because the copy and paste areas aren't the same size.")); return; } for(int i=0; i< numRows; i++){ QStringList columns = rows[i].split('\t'); for(int j=0; j<numColumns; j++){ int row = range.topRow() + i; int column = range.leftColumn() + j; if(row < RowCount && column < ColumnCount) setFormula(row, column, columns[j]); } } somethingChanged(); } |
MainWindowクラスから利用するための定義をします。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 | class MainWindow : public QMainWindow { Q_OBJECT public: MainWindow(); protected: void closeEvent(QCloseEvent *event); private slots: void cut(); private: QAction *deleteAction; QAction *cutAction; QAction *copyAction; QAction *deleteAction; } void MainWindow::cut() { spreadsheet->cut(); } void MainWindow::createActions() { cutAction = new QAction(tr("&Cut"),this); // cutAction->setIcon(QIcon(":/images/cut.png")); cutAction->setShortcut(tr("Ctrl+X")); cutAction->setStatusTip(tr("cut the selected cell")); connect(cutAction, SIGNAL(triggered()), this, SLOT(cut())); copyAction = new QAction(tr("&Copy"),this); // copyAction->setIcon(QIcon(":/images/copy.png")); copyAction->setShortcut(tr("Ctrl+C")); copyAction->setStatusTip(tr("copy the selected cell")); connect(copyAction, SIGNAL(triggered()), this, SLOT(copy())); delAction = new QAction(tr("&Delete"),this); // delAction->setIcon(QIcon(":/images/delete.png")); delAction->setShortcut(tr("Del")); delAction->setStatusTip(tr("delete the selected cell")); connect(delAction, SIGNAL(triggered()), this, SLOT(del())); pasteAction = new QAction(tr("&Paste"),this); // pasteAction->setIcon(QIcon(":/images/paste.png")); pasteAction->setShortcut(tr("Ctrl+V")); pasteAction->setStatusTip(tr("paste the copied cell")); connect(pasteAction, SIGNAL(triggered()), this, SLOT(paste())); } void MainWindow::createMenus() { editMenu = menuBar()->addMenu(tr("&Edit")); selectMenu = menuBar()->addMenu(tr("&Select")); editMenu->addSeparator(); editMenu->addAction(cutAction); editMenu->addAction(copyAction); editMenu->addAction(pasteAction); editMenu->addAction(delAction); } |