Qtプログラミング – スプレッドシート検索機能実装
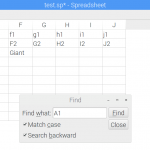
津路です。
前回に引き続き、FindDialogクラスを、mainwindowクラスで利用します。
今回は、スプレッドシートクラスに、前方検索と後方検索を実装します。
以下、ヘッダファイルです。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | #ifndef SPREADSHEET_H #define SPREADSHEET_H #include <QTableWidget> class Cell; class SpreadsheetCompare; class Spreadsheet : public QTableWidget { Q_OBJECT public: Spreadsheet(QWidget *parent = 0); void clear(); public slots: void findNext(const QString &str, Qt::CaseSensitivity cs); void findPrevious(const QString &str, Qt::CaseSensitivity cs); signals: void modified(); private slots: private: Cell *cell(int, int) const; QString text(int, int) const; }; #endif |
以下、実装ファイルです。
前方検索では、選択中のセルより先を検索対象にします。
後方検索では、逆に、選択中のセルより前を検索対象にします。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 | void Spreadsheet::findNext(const QString &str, Qt::CaseSensitivity cs) { int row = currentRow(); int column = currentColumn()+1; while(row < RowCount) { while(column < ColumnCount) { if(text(row,column).contains(str,cs)) { clearSelection(); setCurrentCell(row,column); activateWindow(); return; } column++; } column=0; row++; } QApplication::beep(); } void Spreadsheet::findPrevious(const QString &str, Qt::CaseSensitivity cs) { int row = currentRow(); int column = currentColumn()-1; while(row >=0) { while(column >=0) { if(text(row,column).contains(str,cs)) { clearSelection(); setCurrentCell(row,column); activateWindow(); return; } column--; } column=ColumnCount-1; row--; } QApplication::beep(); } |
これらの関数を利用します。
1 2 3 4 5 6 7 8 9 10 | void MainWindow::find() { if(!findDialog) { findDialog = new FindDialog(this); connect(findDialog, SIGNAL(findNext(const QString &, Qt::CaseSensitivity)), spreadsheet, SLOT(findNext(const QString &, Qt::CaseSensitivity))); connect(findDialog, SIGNAL(findPrevious(const QString &, Qt::CaseSensitivity)), spreadsheet, SLOT(findPrevious(const QString &, Qt::CaseSensitivity))); } findDialog->show(); findDialog->activateWindow(); } |