ラズパイデスクトップでPyQt5入門(7)GridLayout,Review example
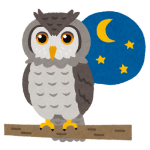
こんばんは。國松です。
今回はPyQt5のLayout Management の続きです。 GridLayoutとReview exampleを見ていきます。
参考サイト
ZetCode PyQt5 tutorial
【PythonでGUI】PyQt5 -始めの一歩-
3.GridLayout
QGridLayoutはスペースを行と列に分割します。今回は格子状にボタンを配置して電卓(見た目だけ)を作成していきます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 | #!/usr/bin/python3 #coding: utf-8 """ In this example, we create a skeleton of a calculator using QGridLayout. """ import sys from PyQt5.QtWidgets import (QWidget, QGridLayout, QPushButton, QApplication) class Example(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): #アプリケーション画面のためのオブジェクトを作成 grid = QGridLayout() self.setLayout(grid) #1ボタンのラベル name = ['Cls', 'Bck', '', 'Close', '7', '8', '9', '/', '4', '5', '6', '*', '1', '2', '3', '-', '0', '.', '=', '+'] #ボタンの位置を設定 positions = [(i,j) for i in range(5) for j in range(4)] #画面にボタンを追加 for position, name in zip(positions, name): #ボタンのラベルがないときは次へ if name == '': continue #ボタンのラベルを設定 button = QPushButton(name) #ボタンを*positionの位置に配置 grid.addWidget(button, *position) self.move(300,150) self.setWindowTitle('Calculator') self.show() if __name__ == '__main__': app = QApplication(sys.argv) ex = Example() sys.exit(app.exec_()) |
GridLayoutを使ってボタンが綺麗に配置されました。
4.Review example(テキストボックスのレイアウト)
ウィジェットはグリッド内の複数の列または行にまたがることができます。次の例ではそれを示していきたいと思います。
3つのラベル、2つの行編集、1つのテキスト編集ウィジェットがあるウィンドウを作成します。 レイアウトはQGridLayoutで行われます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 | #!/usr/bin/python3 #coding: utf-8 """ In this example, we create a bit more complicated window layout using the QGridLayout manager """ import sys from PyQt5.QtWidgets import (QWidget, QLabel, QLineEdit, QTextEdit, QGridLayout, QApplication) class Example(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): title = QLabel('Title') author = QLabel('Author') review = QLabel('Review') titleEdit = QLineEdit() authorEdit = QLineEdit() reviewEdit =QTextEdit() #グリッドレイアウトを作成し各ウィジェットの間隔を設定 grid = QGridLayout() grid.setSpacing(10) grid.addWidget(title,1,0) #入力欄の位置設定 grid.addWidget(titleEdit,1,1) grid.addWidget(author, 2,0) grid.addWidget(authorEdit,2,1) grid.addWidget(review, 3,0) #Reviewウィジェットを5行に広げる grid.addWidget(reviewEdit,3,1,5,1) self.setLayout(grid) self.setGeometry(300,300,350,300) self.setWindowTitle('Review') self.show() if __name__ == '__main__': app = QApplication(sys.argv) ex = Example() sys.exit(app.exec_()) |
日本語も入力できるみたいですね。次回はPyQt5のEventとSingalについて見ていきたいと思います。