ラズパイデスクトップでPyQt5入門(2)
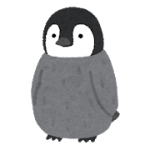
こんばんは。國松です。
PyQt5入門の2回目はtooltipの作成から始めたいと思います。
参考サイト
ZetCode PyQt5 tutorial
【PythonでGUI】PyQt5 -始めの一歩-
3.Showing a Tooltip
tooltipとはマウスオーバーしたときに表示される枠内の補足説明などの事です。
今回はwindowとボタンに対するtooltipを作成していきます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 | #!/usr/bin/python3 # coding: utf-8 import sys from PyQt5.QtWidgets import(QWidget, QToolTip,QPushButton,QApplication) from PyQt5.QtGui import QFont class Example(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): #10pxのサンセリフォントを使う QToolTip.setFont(QFont('SanSserif',10)) #画面の吹き出し設定 self.setToolTip('This is a <b>QWidget</b> widget') #ボタンを作る btn = QPushButton('Button',self) #ボタンの吹き出し設定 btn.setToolTip('This is a <b>QPushButton</b> widget') #ボタンのサイズを自動設定 btn.rerize(btn.sizeHint()) #ボタンの位置を設定 btn.move(50,50) self.setGeometry(300,300,300,200) self.setWindowTitle('ToolTip') self.show() if __name__ == '__main__': app = QApplication(sys.argv) ex = Example() sys.exit(app.exec_()) |
4.Closing a Window
画面(window)を閉じるボタンを作成していきます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 | #!/usr/bin/python3 # coding: utf-8 import sys from PyQt5.QtWidgets import(QWidget, QToolTip,QPushButton,QApplication) from PyQt5.QtGui import QFont class Example(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): #10pxのサンセリフォントを使う QToolTip.setFont(QFont('SanSserif',10)) #画面の吹き出し設定 self.setToolTip('This is a <b>QWidget</b> widget') #ボタンを作る btn = QPushButton('Button',self) #ボタンの吹き出し設定 btn.setToolTip('This is a <b>QPushButton</b> widget') #ボタンのサイズを自動設定 btn.rerize(btn.sizeHint()) #ボタンの位置を設定 btn.move(50,50) self.setGeometry(300,300,300,200) self.setWindowTitle('ToolTip') self.show() if __name__ == '__main__': app = QApplication(sys.argv) ex = Example() sys.exit(app.exec_()) |
5.MessageBox
MessageBoxを作成して画面を閉じる時の確認画面を表示していきたいと思います。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 | #!/usr/bin/python3 # -*-coding: utf^8 -*- """ This program shows a confirmation message box when we click on the close button of the application window. """ import sys from PyQt5.QtWidgets import QWidget, QMessageBox, QApplication class Example(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): self.setGeometry(300, 300, 250, 150) self.setWindowTitle('Message box') self.show() def closeEvent(self, event): reply = QMessageBox.question(self, 'Message', "Are you sure to quit?", QMessageBox.Yes | QMessageBox.No, QMessageBox.No) if reply == QMessageBox.Yes: event.accept() else: event.ignore() if __name__ == '__main__': app = QApplication(sys.argv) ex = Example() sys.exit(app.exec_()) |
おまけ
QuitButoonとMessageBoxを組み合わせます。QuitButtonを押しても画面のタイトルバーの✖を押してもMessageBoxが表示されます。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 | #!/usr/bin/python3 #codinf: utf-8 import sys from PyQt5.QtWidgets import QWidget,QMessageBox, QPushButton, QApplication class Example(QWidget): def __init__(self): super().__init__() self.initUI() def initUI(self): qbtn = QPushButton('Quit', self) qbtn.clicked.connect(self.button_quit) qbtn.resize(qbtn.sizeHint()) qbtn.move(50,50) self.setGeometry(300, 300, 250, 150) self.setWindowTitle('Message box') self.show() def button_quit(self): # self.statusBar().showMessage('Leaving Application') self.close() def closeEvent(self, event): reply = QMessageBox.question(self, 'Message', "Are you sure to quit?",QMessageBox.Yes | QMessageBox.No,QMessageBox.No) if reply == QMessageBox.Yes: event.accept() else: event.ignore() if __name__ == '__main__': app = QApplication(sys.argv) ex = Example() sys.exit(app.exec()) |